Unit Tests vs Integration Tests vs Functional Test: Which One to Choose?
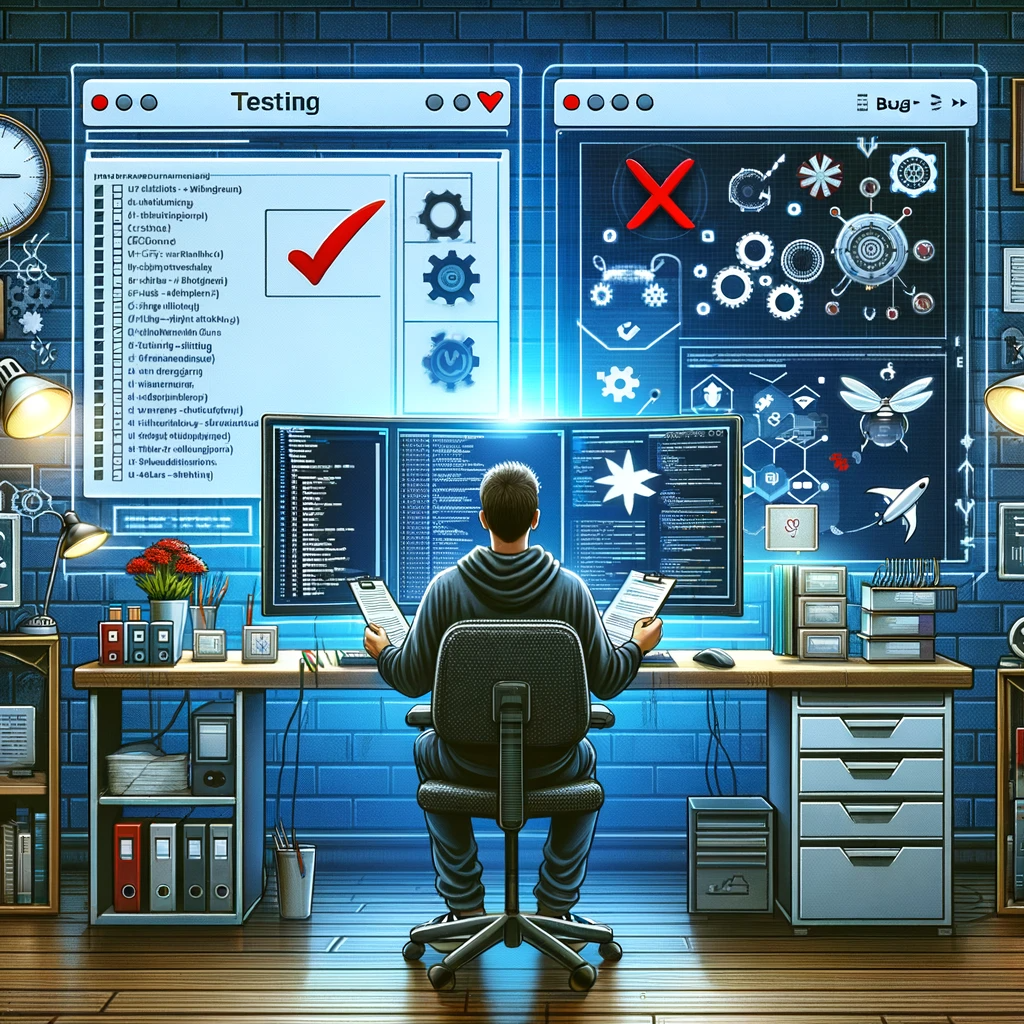
In software development, testing is a critical phase that ensures the functionality, reliability, and efficiency of your application. There are various types of tests performed at different levels of development; among them are unit tests, integration tests and functional tests. They all play crucial roles in building robust applications but serve distinct purposes.
The different software testing strategies
- Unit Tests: These are the most granular form of testing, focused on individual components or functions of an application. The objective is to validate that each part performs as expected in isolation. They are quick to execute and are typically automated.
- Integration Tests: These tests assess the interaction and integration between different modules or parts of the application. The goal is to ensure that combined components function together properly. Integration tests are more complex than unit tests and often require additional setup like configuring databases or network connections.
- Functional Tests: This testing is concerned with the overall functionality of the application from an end-user perspective. It involves testing complete features or user journeys to ensure the application behaves as intended. These tests can be automated or manual and often simulate user interactions with the application.
Understanding the Purpose and Benefits of Unit Tests
Unit testing involves verifying individual components or 'units' within an application to ensure they work as intended independently. These units could be methods, classes, or functions that carry out specific tasks within your codebase. The goal here is to isolate each part of the program and verify its correctness.
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(-1, -1), -2)
if __name__ == '__main__':
unittest.main()
Benefits of Unit Testing
Early bug detection: Unit tests help in detecting bugs early in the development cycle, making it easier and less costly to fix them.
Improved code quality: Writing unit tests often leads to better-designed, modular, and maintainable code.
Regression testing: Unit tests can be run quickly and frequently, ensuring that new changes do not break existing functionality.
Faster debugging: When a test fails, it is easier to pinpoint the cause of the failure within a specific unit rather than through manual testing of the entire application.
Documentation: Unit tests serve as living documentation that demonstrates how each part of the code should behave.
Drawbacks of Unit Testing
Time-consuming: Writing comprehensive unit tests can add overhead to development time, especially for complex systems or legacy code without proper test coverage.
False sense of security: Passing unit tests do not guarantee that there are no integration issues or higher-level problems within the system.
Maintenance overhead: As the codebase evolves, maintaining and updating existing unit tests can become cumbersome if not properly managed.
Testing only known paths: Developers may unintentionally write tests based on their assumptions about how a component should work, potentially missing edge cases or unexpected behaviors.
While there are significant benefits to incorporating unit testing into software development practices, it's essential to weigh these against potential drawbacks such as time investment and maintenance overhead in order to determine its overall value for a particular project or organization.
Advantages and Challenges of Integration Testing in Software Development
Integration testing is an essential part of the software development process, as it helps ensure that different components of a system work together as intended.
import unittest
import requests
class TestAddAPIIntegration(unittest.TestCase):
def test_add_api(self):
response = requests.get('http://localhost:5000/add', params={'a': 2, 'b': 3})
self.assertEqual(response.status_code, 200)
self.assertEqual(response.json(), {'result': 5})
if __name__ == '__main__':
unittest.main()
Benefits of Integration Tests
Comprehensive Testing: Integration tests evaluate the interactions between various modules or components within a system, providing comprehensive coverage that can uncover issues related to data flow, communication protocols, and dependencies.
Realistic Scenarios: By testing multiple interconnected parts of a system simultaneously, integration tests simulate real-world usage scenarios more accurately than unit tests alone. This can reveal potential problems that may only arise during actual system operation.
Detecting Interface Issues: Integration tests are effective at identifying interface mismatches or compatibility issues between different modules or services, helping teams address these challenges early in the development lifecycle.
Improved Confidence in System Behavior: Successful integration testing instills confidence in the overall behavior and performance of a software application by verifying that its integrated components function correctly together.
Reduced Debugging Effort: Identifying defects at the integration level can reduce debugging efforts later in the development cycle by catching issues before they propagate through multiple layers of code.
Cons of Integrations Tests
Complexity and Setup Overhead: Writing integration test cases often requires setting up complex environments with all dependent systems running properly – leading to increased setup time compared to unit testing.
Execution Time and Cost: Due to their broader scope, integration tests typically take longer to execute than unit tests; this could result in higher resource consumption (e.g., time & cost) for running automated test suites regularly.
Difficulty Isolating Failures: When an issue arises during an integration test run, pinpointing its exact cause among numerous interacting elements might be challenging – potentially complicating troubleshooting efforts for developers.
Maintenance Challenges: As systems evolve over time with new features added or existing ones modified, maintaining large sets of intricate integration test cases becomes increasingly burdensome without proper organization strategies.
Fragile Test Environment Dependencies:
- External dependencies such as databases or APIs introduce fragility into integrations due to potential changes outside your control.
- Test environment stability relies on consistent availability/accessibility from external resources which may not always be guaranteed.
While there are clear benefits associated with conducting thorough integration testing throughout software development projects—such as improved fault detection capabilities—it's important for teams to carefully consider trade-offs like complexity overheads & maintenance effort against expected gains before incorporating them into their quality assurance strategy. By understanding both sides' perspectives on integrating test practices effectively within workflows while mitigating inherent downsides wherever possible will help strike an optimal balance toward delivering robust solutions fit-for-purpose.
A Look at Functional Tests
Functional testing (or end-to-end testing) takes a more holistic approach where the system is tested against functional requirements/specifications ensuring all parts work seamlessly together under varying scenarios. This type of test checks if your software behaves as expected from an end-user's perspective.
from selenium import webdriver
import unittest
class TestWebAppFunctionality(unittest.TestCase):
def setUp(self):
self.browser = webdriver.Chrome()
def test_form_submission(self):
self.browser.get('http://localhost:8000') # URL of the web app
input1 = self.browser.find_element_by_id('input1')
input2 = self.browser.find_element_by_id('input2')
submit_button = self.browser.find_element_by_id('submit')
input1.send_keys('2')
input2.send_keys('3')
submit_button.click()
result = self.browser.find_element_by_id('result').text
self.assertEqual(result, '5')
def tearDown(self):
self.browser.quit()
if __name__ == '__main__':
unittest.main()
The Advantages of Functional Testing
Validation of user requirements: Functional testing ensures that the software meets specified business requirements and functions as intended by the end users.
End-to-end validation: It tests the entire system or specific features to ensure they work together as expected, providing confidence in overall system functionality.
Regression testing: Functional tests can be reused for regression testing to verify that new changes do not introduce unintended side effects or break existing functionality.
Customer satisfaction: By validating key functionalities, functional testing contributes to delivering a reliable and high-quality product, which can enhance customer satisfaction.
The Disadvantages of Function Testing
Time-consuming: Writing comprehensive functional test cases for complex systems or large applications may require significant time and effort.
Limited scope: While functional tests validate whether an application meets its specifications, they may not cover non-functional aspects such as performance, security, or usability.
Maintenance overhead: As the application evolves, maintaining and updating existing functional test cases can become challenging if not properly managed.
Dependencies on environment and data: Functional tests often rely on specific environments and data sets, making them sensitive to changes in these factors.
While functional testing plays a crucial role in ensuring that an application meets its intended functionality requirements, it's important to consider potential drawbacks such as time investment and maintenance overhead when determining its value within a broader quality assurance strategy for software development projects.
The Preference for Integration Tests
While unit, integration and functional tests are essential in different stages of development, leaning more towards integration testing will provide better returns.
As product development can rapidly change and evolved, Integration Tests will ease your development process and ensure quick delivery without impacting reliability.
By prioritizing integration testing, organizations can achieve comprehensive validation while optimizing resources, ultimately leading to improved software quality, cost-effectiveness and an overall reliability and robustness of software systems.