Pydantic: Simplify Your Python Development Process
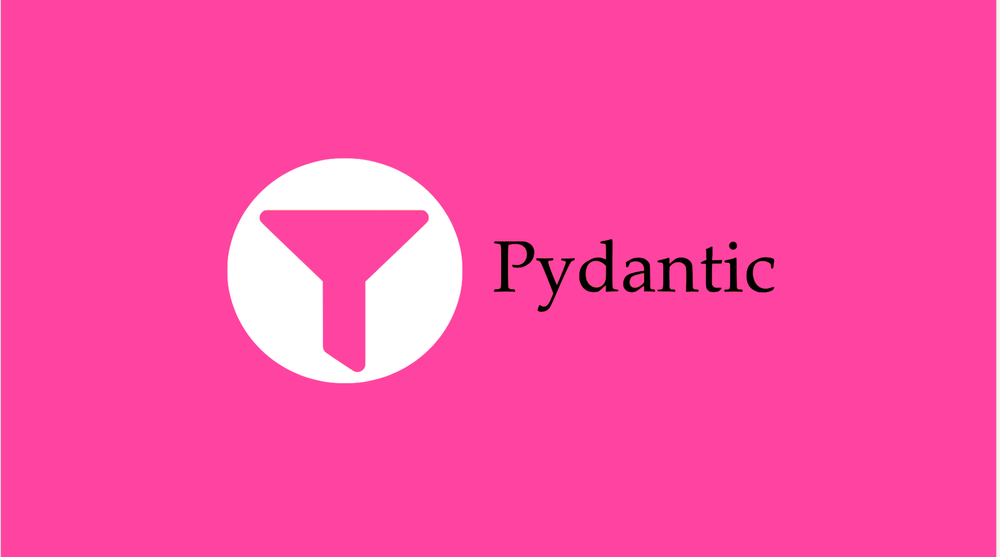
Hello there, fellow developers!
In today's blog post, we are going to dive into the world of Pydantic. If you've never heard of Pydantic before, don't worry; I'm here to introduce you to this incredible library that will simplify your Python development process.
What is Pydantic?
Pydantic is a fantastic open-source library for data validation and settings management in Python applications. It provides a way to define how data should be structured and validated using Python type hints, annotations, and powerful runtime checks.
With Pydantic, you can easily create simple yet highly scalable models with minimal effort. This means fewer bugs and better readability of your codebase – it's truly a win-win situation!
Main Features
Type Validation
Pydantic's core feature is its ability to perform rigorous type validation. It empowers developers to enforce strict typing conventions within their applications.
With the use of Python's built-in Type Hints, Pydantic ensures that your data adheres to the specified data types. This helps maintain data integrity and reliability throughout your code.
from pydantic import BaseModel
class Person(BaseModel):
name: str
age: int
# Creating an instance of the Pydantic model with type validation
person_data = Person(name="Alice", age=30)
# This will raise a validation error since 'age' is not an integer
# person_data = Person(name="Bob", age="Not an integer")
Data Serialization
Beyond type validation, Pydantic excels in data serialization. It simplifies the process of transforming complex data structures into compatible formats.
Whether you're working with external systems, databases, or APIs, Pydantic streamlines data interchange, making integration seamless.
from pydantic import BaseModel
class Book(BaseModel):
title: str
author: str
book_data = {"title": "Python for Beginners", "author": "John Doe"}
# Deserialize JSON data into a Pydantic model
book = Book(**book_data)
print(book.title) # Output: Python for Beginners
# Serialize Pydantic model to JSON
book_json = book.json()
print(book_json) # Output: {"title": "Python for Beginners", "author": "John Doe"}
Model Validation
Pydantic enables you to create data models with validation rules. You can define constraints, default values, and even implement custom validation functions for each field in your data model.
This ensures that your data remains consistent and meets your specific requirements.
from pydantic import BaseModel, validator
class Person(BaseModel):
name: str
age: int
@validator("age")
def validate_age(cls, value):
if value < 0 or value > 120:
raise ValueError("Age must be between 0 and 120")
return value
# Creating an instance of the Pydantic model with validation
person_data = Person(name="Alice", age=25)
# This will raise a validation error due to age being out of range
# person_data = Person(name="Bob", age=150)
Parsing and Parsing Defaults
Pydantic makes parsing data from various sources a breeze. It provides straightforward methods for parsing data from sources like JSON, forms, and query parameters.
Additionally, you can set default values for fields when data is missing or invalid, enhancing data handling robustness.
from pydantic import BaseModel, Field
class Item(BaseModel):
name: str
price: float = Field(..., description="Price of the item", gt=0)
# Parsing JSON data into a Pydantic model
item_data = {"name": "Widget", "price": 10.99}
item = Item(**item_data)
# Setting default values for missing fields
default_item = Item(name="Default Widget")
Nested Models
Dealing with complex, hierarchical data structures? Pydantic has you covered.
You can create nested Pydantic models within each other, making it easy to handle deeply nested data with ease and clarity.
from pydantic import BaseModel
class Address(BaseModel):
street: str
city: str
class Person(BaseModel):
name: str
age: int
address: Address
# Creating a nested Pydantic model
person_data = {
"name": "Alice",
"age": 30,
"address": {"street": "123 Main St", "city": "Exampleville"}
}
person = Person(**person_data)
Field Aliases
Pydantic supports field aliases, allowing you to define multiple names for a single field.
This feature proves invaluable when working with data from various sources that may use different naming conventions.
from pydantic import BaseModel, Field, root_validator
class Item(BaseModel):
name: str
price: float = Field(..., alias="item_price")
@root_validator(pre=True)
def set_alias(cls, values):
values["price"] = values.pop("item_price")
return values
item_data = {"name": "Widget", "item_price": 10.99}
item = Item(**item_data)
print(item.price) # Output: 10.99
Custom Validators
For precise control over data validation, Pydantic allows you to define custom validation functions for fields.
These custom validators enable you to implement specific business logic to ensure data correctness.
from pydantic import BaseModel, validator
class Item(BaseModel):
name: str
price: float
@validator("price")
def validate_price(cls, value):
if value < 0:
raise ValueError("Price cannot be negative")
return round(value, 2)
item_data = {"name": "Widget", "price": -5.6789}
item = Item(**item_data)
Async Model Validation
In the world of asynchronous Python, Pydantic shines by supporting asynchronous model validation.
This feature is especially useful when working with async frameworks and APIs, ensuring your data remains valid even in asynchronous scenarios.
Documentation Generation
Last but not least, Pydantic can automatically generate documentation for your data models.
This feature helps in understanding and using your data structures, making your code more transparent and accessible.
Integration with FastAPI
If you are already familiar with FastAPI, you'll be thrilled to know that Pydantic integrates seamlessly with it.
With FastAPI's automatic request validation and response serialization capabilities integrated with Pydantic models, building robust and highly performant web APIs becomes a breeze.
Using Pydantic models in your FastAPI applications allows you to define data structures for input payloads and output responses. It also helps in automatically generating interactive API documentation using tools like Swagger UI or ReDoc.
Here is an example:
from fastapi import FastAPI
from pydantic import BaseModel
app = FastAPI()
class Item(BaseModel):
name: str
description: str | None = None
price: float
tax: float | None = None
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item):
results = {"item_id": item_id, "item": item}
return results
Conclusion
In conclusion, Pydantic is an excellent library that brings simplicity and robustness to your Python development process. With its easy-to-use API and seamless integration with FastAPI, it becomes even more powerful.
I encourage you all to give Pydantic a try in your next project. Trust me; you won't regret it!